Welcome to our article on bubble sort, a simple sorting algorithm that is widely used for sorting lists and arrays. In this section, we will explore the basics of bubble sort, its efficiency, and how it compares to other sorting algorithms. So let’s dive in and understand how bubble sort works!
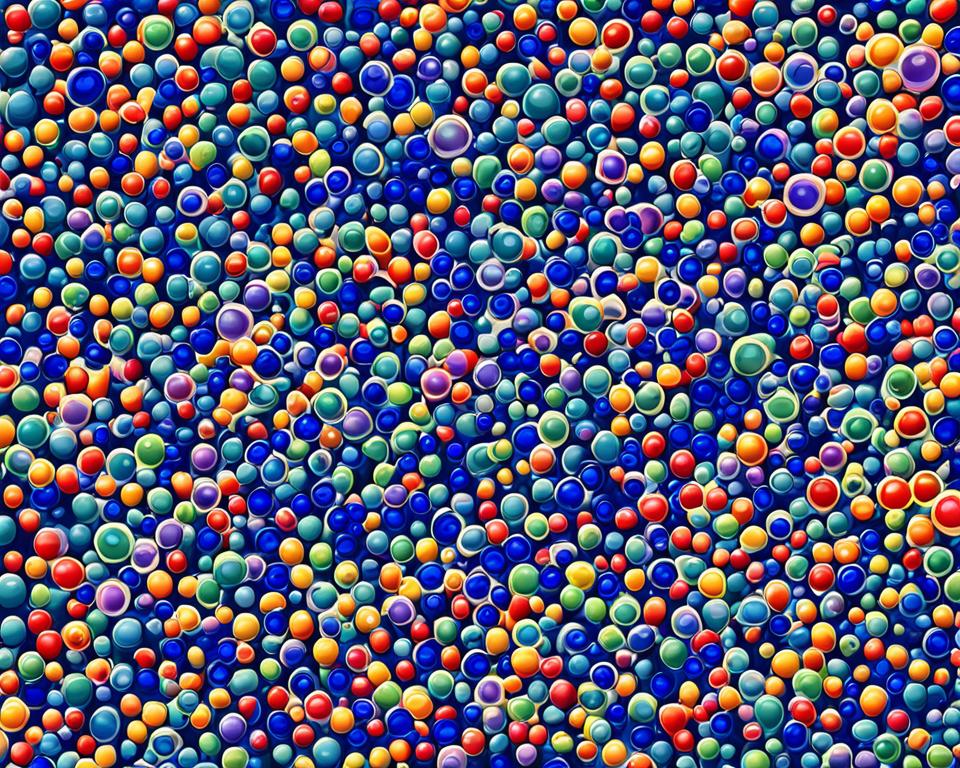
Key Takeaways:
- Bubble sort is a simple comparison-based sorting algorithm.
- It works by repeatedly comparing adjacent elements and swapping them if they are in the wrong order.
- The algorithm continues this process until the list is sorted.
- While bubble sort is easy to understand and implement, it is not efficient for large datasets due to its time complexity.
- Bubble sort has a space complexity of O(1), meaning it operates directly on the input list without requiring additional memory.
What is Bubble Sort and How Does it Work?
Bubble sort is a simple comparison-based sorting algorithm that is widely used in computer science. It operates by repeatedly comparing adjacent elements in a list and swapping them if they are in the wrong order. This process continues until the entire list is sorted.
The algorithm gets its name from the way smaller elements “bubble” their way to the beginning of the list, while larger elements gradually move towards the end.
The simplicity of bubble sort makes it an excellent choice for teaching basic sorting concepts. It is easy to understand and implement, making it accessible even for novice programmers. While bubble sort is not the most efficient sorting algorithm, it serves as a good starting point for understanding more complex sorting algorithms.
Bubble sort belongs to the family of comparison-based sorting algorithms, which means it relies on comparing elements to determine their order. Each pair of adjacent elements is compared using a comparison operator, and if they are out of order, their positions are swapped. This process continues until the entire list is sorted.
Due to its simplicity, bubble sort has a time complexity of O(n^2) in the worst-case scenario, where n represents the number of elements in the list. This makes bubble sort less efficient compared to other sorting algorithms when dealing with large datasets.
However, bubble sort has its advantages. It is an in-place sorting algorithm, meaning that it does not require additional memory beyond the initial list. Additionally, bubble sort is a stable sorting algorithm, which means that elements with the same key value maintain their relative order in the sorted output.
Bubble Sort in Computer Programming
In computer programming, bubble sort is a popular choice for teaching new programmers the fundamentals of sorting data sets. Its simplicity and ease of implementation make it an ideal algorithm to introduce beginners to the concept of sorting.
Although bubble sort is not the most efficient algorithm for sorting large datasets due to its time complexity, it excels in sorting small datasets or datasets that are already mostly sorted. The algorithm’s straightforward nature allows programmers to grasp the basic principles of sorting and gain hands-on experience in implementing a basic sorting algorithm.
By using bubble sort, programmers can develop their understanding of how sorting algorithms work and learn important concepts like adjacent element comparison and swapping. This algorithmic knowledge forms the foundation for exploring more advanced and efficient sorting techniques.
Sorting algorithms not only sort data but also help for using with searching algorithms such as binary search which is an algorithm that has a time complexity of O(log N) in the worst case. As a prerequisite in binary search, the data should be sorted. Therefore, this kind of an algorithm helps in such kind of a scenario.
When dealing with small datasets or nearly sorted datasets, bubble sort can still be a reliable choice. Its simplicity and intuitive logic make it a valuable tool for quick sorting tasks that require basic sorting capabilities. Programmers can use bubble sort to improve the organization and presentation of data in their applications, enhancing the overall user experience.
In computer programming, having a solid understanding of bubble sort opens the door to exploring more complex sorting algorithms and understanding their time and space complexities. By starting with bubble sort, programmers can build a strong foundation in sorting concepts that will prove invaluable as they tackle more challenging sorting problems in the future.
Use Case : Bubble Sort for Product Managers
As product managers, they often find themselves faced with the challenge of prioritizing their work and making decisions about where to allocate their limited time and resources. This is where bubble sort, a simple and intuitive sorting algorithm, can come in handy.
Similar to how bubble sort arranges a string of numbers in the correct order, product managers can use bubble sort as a methodology to prioritize competing initiatives. By evaluating each initiative and comparing them against others, they can determine their relative importance and urgency.
Just like how bubble sort examines adjacent elements and switches their positions if they are out of order, they can evaluate the urgency of each initiative and prioritize them accordingly. They can consider factors such as customer impact, strategic alignment, and resource availability to determine the correct order in which to tackle their projects.
Using bubble sort allows them to approach prioritization in a methodical and structured manner. It provides a transparent and efficient way to analyze their options and make informed decisions based on their limited time and resources.
With bubble sort, they can ensure that their team focuses on the most critical initiatives first, maximizing the impact of their work and minimizing the risk of wasting valuable resources on less important tasks.
Other Sorting Algorithms
Bubble sort is just one of many sorting algorithms available. When it comes to sorting data, there are various options to choose from, each with its own advantages and disadvantages.
Here are some other popular sorting algorithms:
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
- Heap Sort
- Bucket Sort
Each of these algorithms has its unique characteristics and is suitable for different scenarios. The choice of algorithm depends on the specific requirements of the sorting task at hand.
How Bubble Sort Works
Bubble sort is a simple and intuitive sorting algorithm that operates by repeatedly comparing adjacent elements in a list and swapping them if they are in the wrong order. The algorithm starts from the beginning of the list and continues until it reaches the end without any swaps. This process is repeated multiple times until the list is sorted in the correct order.
The key concept behind bubble sort is that with each iteration, the largest (or smallest, depending on the sorting order) element “bubbles” to the top of the list. This is accomplished by comparing adjacent elements and swapping them if they are not in the correct order. By repeatedly performing these comparisons and swaps, the algorithm gradually moves the largest element towards the end of the list.
Let’s take a visual example to better understand how bubble sort works:
In the image above, we have an unsorted array of numbers [5, 2, 8, 3, 1, 6]. The adjacent pairs of elements are compared and swapped until the list is sorted in ascending order. The process can be broken down into the following steps:
- Step 1: [5, 2, 8, 3, 1, 6] → [2, 5, 8, 3, 1, 6]
- Step 2: [2, 5, 8, 3, 1, 6] → [2, 5, 3, 8, 1, 6]
- Step 3: [2, 5, 3, 8, 1, 6] → [2, 5, 3, 1, 8, 6]
- Step 4: [2, 5, 3, 1, 8, 6] → [2, 5, 3, 1, 6, 8]
- Step 5: [2, 5, 3, 1, 6, 8] → [2, 3, 5, 1, 6, 8]
- Step 6: [2, 3, 5, 1, 6, 8] → [2, 3, 1, 5, 6, 8]
- Step 7: [2, 3, 1, 5, 6, 8] → [2, 3, 1, 5, 6, 8]
- Step 8: [2, 3, 1, 5, 6, 8] → [2, 1, 3, 5, 6, 8]
- Step 9: [2, 1, 3, 5, 6, 8] → [1, 2, 3, 5, 6, 8]
At the end of the process, the list is sorted in the correct order: [1, 2, 3, 5, 6, 8]. Bubble sort works by repeatedly comparing adjacent elements and swapping them until the list is sorted, ensuring that each iteration brings the correct order closer to completion.
Advantages of Bubble Sort
- Simple and intuitive algorithm, easy to understand and implement.
- In-place sorting, does not require additional memory.
- Stable sorting algorithm, maintains the relative order of elements with the same value.
Disadvantages of Bubble Sort
- Inefficient for large datasets due to its time complexity of O(N^2).
- Relies on comparison operators, limiting its efficiency in certain scenarios.
Implementing Bubble Sort in Different Programming Languages
Bubble sort, a simple and popular sorting algorithm, can be implemented in various programming languages, including C++, Java, Python, PHP, and JavaScript. While each language may have its own syntax and specific implementation details, the basic logic of the bubble sort algorithm remains the same.
Let’s take a look at how bubble sort can be implemented in some of these programming languages:
Python
In Python, bubble sort can be implemented using a while loop and a for loop in a more simpler manner:
C++
Java
PHP
C#
JavaScript
These are just a few examples showcasing the implementation of bubble sort in different programming languages. The logic behind bubble sort remains the same regardless of the language used, making it a versatile algorithm for sorting arrays and lists.
Time Complexity of Bubble Sort
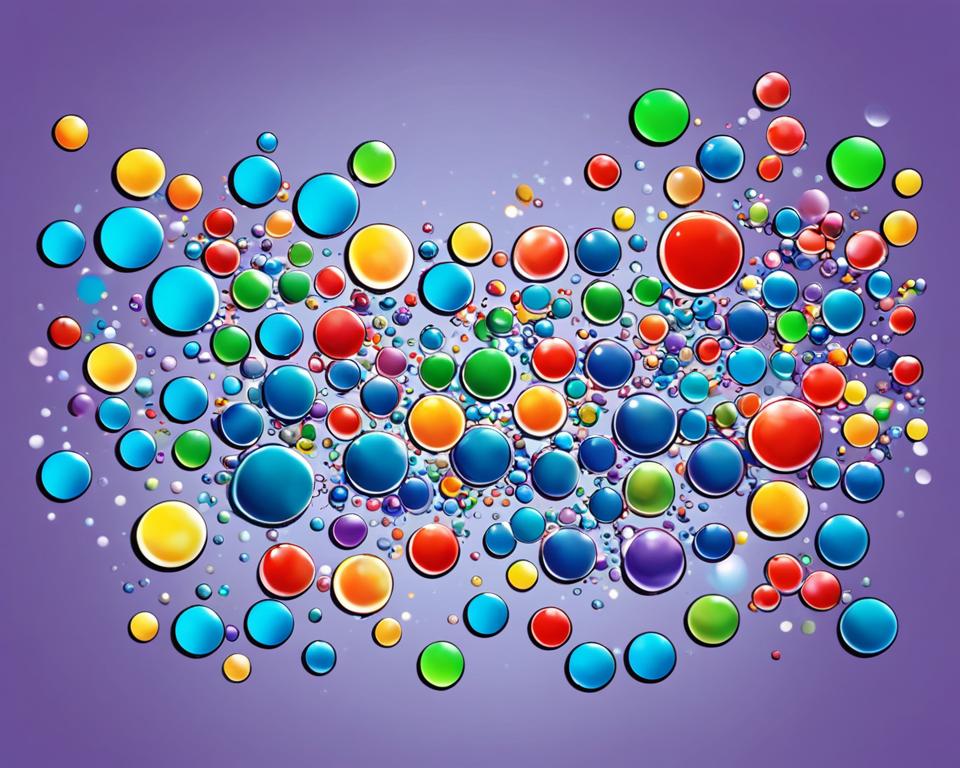
In the world of sorting algorithms, time complexity is a critical measure of efficiency. It determines how much time it takes for an algorithm to run based on the input size. When it comes to bubble sort, the time complexity is represented by the Big O notation O(N^2).
The Big O notation is a mathematical representation that expresses the upper bound of the time complexity in relation to the input size. In the case of O(N^2), it means that the time required to sort a list of size N using bubble sort is proportional to N squared.
So what does this mean for bubble sort? In practical terms, it means that as the size of the list increases, the number of comparisons and swaps required also grows exponentially. This makes bubble sort less efficient compared to other sorting algorithms, especially when dealing with large datasets.
Let’s visualize this concept with an example:
- For a list of size 10, bubble sort may require 100 comparisons and swaps.
- For a list of size 100, bubble sort may need 10,000 comparisons and swaps.
- And for a list of size 1000, bubble sort could potentially involve a staggering 1,000,000 comparisons and swaps.
This exponential increase in operations as the list size grows is the main reason why bubble sort is considered inefficient for large datasets. Sorting algorithms with better time complexity, such as merge sort or quicksort, can handle larger inputs more efficiently.
However, it’s worth noting that bubble sort still has its uses. It can be a suitable choice for small datasets or nearly sorted lists, where its simplicity and easy implementation can outweigh the inefficiencies in time complexity. It’s important to consider the specific requirements of your sorting task and choose the appropriate algorithm accordingly.
Now that we understand the time complexity of bubble sort, let’s move on to exploring the space complexity, which will shed light on the memory requirements of this sorting algorithm.
Space Complexity of Bubble Sort
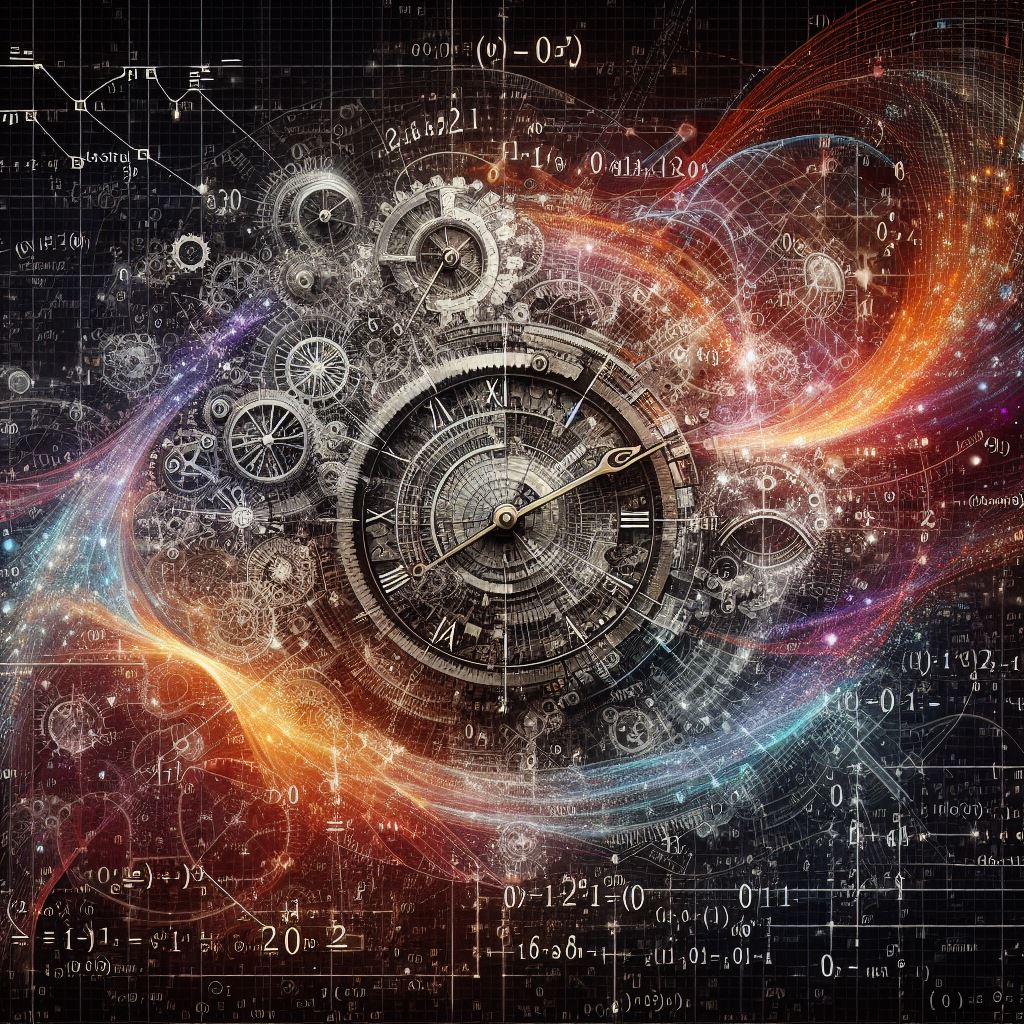
When considering the efficiency of a sorting algorithm like bubble sort, it is essential to analyze its space complexity. The space complexity of an algorithm determines the amount of additional memory it requires to perform its operations. In the case of bubble sort, the space complexity is incredibly efficient, with a constant space requirement of O(1).
Bubble sort is known for its in-place sorting technique, which means it works directly on the input list without utilizing any auxiliary data structures or allocating extra memory. This characteristic is highly advantageous in scenarios where memory usage needs to be minimized or when dealing with large data sets where additional space allocation might be impractical or not feasible.
The in-place nature of bubble sort allows it to efficiently sort the input list by swapping adjacent elements until the entire list is in the correct order. Unlike other sorting algorithms that may require additional memory to create temporary arrays or data structures, bubble sort achieves the sorting process within the existing memory space. This aspect makes bubble sort an optimal choice for situations with limited resources or when memory efficiency is a critical factor.
With its space complexity of O(1), bubble sort proves to be a practical option for sorting tasks that prioritize space optimization over other considerations. Its ability to sort effectively in-place showcases the algorithm’s simplicity and efficiency, reinforcing its relevance in various practical applications.
To help visualize the inner workings of bubble sort, take a look at the following illustration:
The provided image demonstrates the step-by-step process of bubble sort, emphasizing the algorithm’s ability to modify and arrange elements directly within the input list without any significant additional memory requirements. It captures the essence of the in-place sorting technique employed by bubble sort.
Advantages of Bubble Sort
Bubble sort, despite its simplicity, offers several advantages that make it a worthwhile sorting algorithm to consider:
- Easy to understand and implement: Bubble sort’s straightforward logic makes it an excellent choice for teaching sorting concepts to beginners in programming and computer science.
- In-place sorting: Bubble sort operates directly on the input list without requiring any additional memory. This makes it an efficient choice when working with limited resources or when memory allocation is a concern.
- Stability: Bubble sort is a stable sorting algorithm, meaning that elements with the same key value maintain their relative order in the sorted output. This property is essential when sorting datasets where the sequence of equal elements needs to be preserved.
Overall, the simplicity, in-place sorting nature, and stability of bubble sort make it a valuable tool in certain scenarios. Whether you are teaching sorting algorithms, working with limited resources, or require a stable sorting approach, bubble sort can be a reliable choice.
Disadvantages of Bubble Sort
Despite its simplicity, bubble sort has some significant drawbacks that limit its efficiency in certain scenarios. The two main disadvantages of bubble sort are its time complexity and its reliance on comparison-based sorting.
Inefficient for large datasets
Bubble sort has a time complexity of O(N^2), meaning that the time it takes to sort a list of size N is proportional to N squared. This makes the algorithm inefficient for sorting large datasets. As the size of the list increases, the number of comparisons and swaps required grows exponentially, resulting in slower sorting times.
Reliance on comparison-based sorting
Bubble sort is a comparison-based sorting algorithm, which means that it relies on comparison operators to determine the order of elements. This can limit its efficiency in certain scenarios. For example, if the elements being sorted require complex or expensive comparison operations, bubble sort may not be the most efficient choice. Other sorting algorithms, such as merge sort or quick sort, which use more advanced techniques like divide and conquer, can offer better performance in these cases.
Overall, while bubble sort is a simple and straightforward sorting algorithm, its time complexity and reliance on comparison-based sorting make it less suitable for large datasets or scenarios where efficiency is crucial. Programmers and data scientists often opt for more efficient and optimized sorting algorithms when dealing with larger datasets or performance-sensitive applications.
Common Applications of Bubble Sort
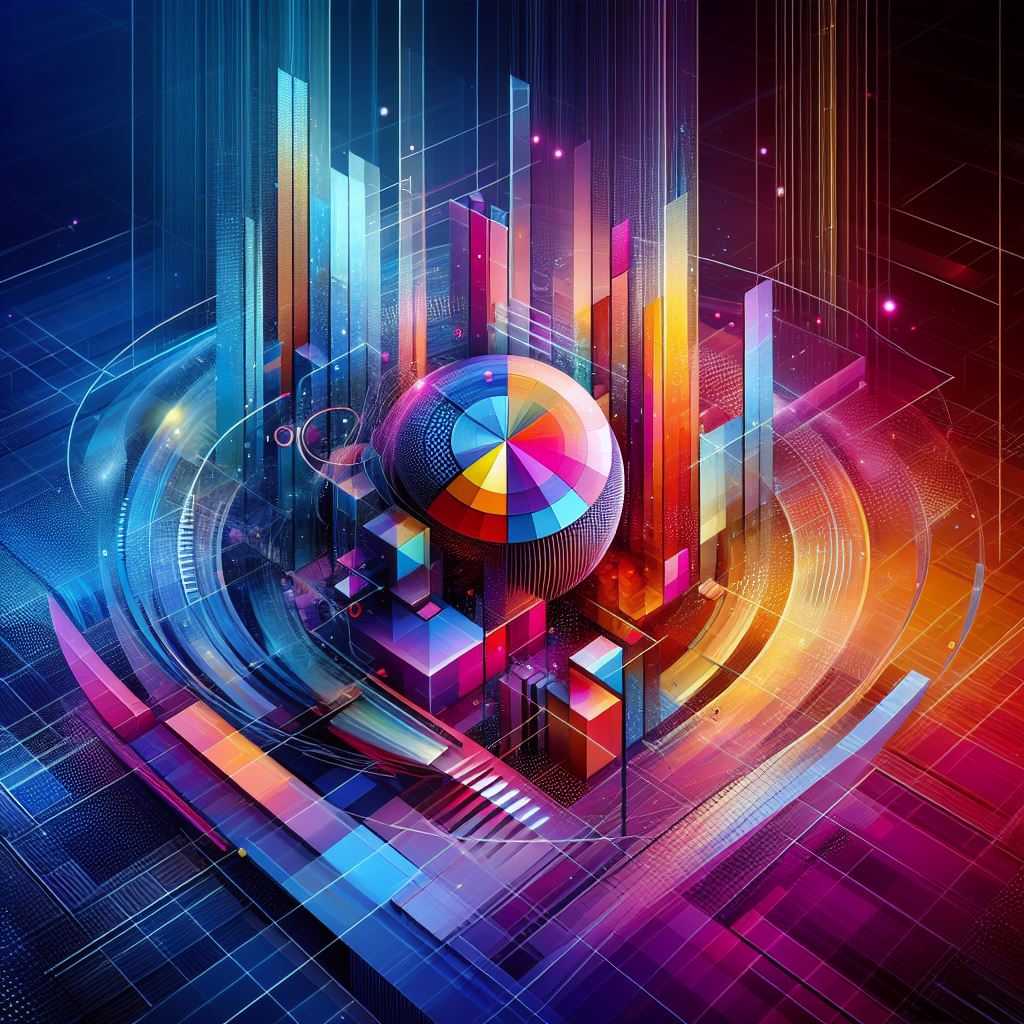
Bubble sort may not be the most efficient sorting algorithm, but it has some practical applications. In computer graphics, it is often used in polygon filling algorithms to detect and fix tiny errors in almost-sorted arrays. Its ability to operate directly on the input data without requiring additional memory makes it suitable for certain scenarios.
- Computer Graphics: Bubble sort plays a crucial role in computer graphics, specifically in polygon filling algorithms. When rendering complex shapes, such as polygons, errors can occur in the sorting of vertices. Bubble sort helps detect and correct these small errors, ensuring accurate rendering and smooth visuals.
While other sorting algorithms may offer better performance, bubble sort’s simplicity and direct manipulation of data make it a viable choice in specific applications where efficiency is not the primary concern. Its straightforward implementation and ability to operate on the input array in-place make it a valuable tool in certain scenarios.
Conclusion
In conclusion, bubble sort is a sorting algorithm that offers simplicity and ease of understanding. While it may not be the most efficient algorithm for large datasets, it still has its value in certain scenarios. Bubble sort is especially useful for teaching basic sorting concepts and for sorting small or nearly sorted datasets.
Although bubble sort’s time complexity of O(N^2) makes it less suitable for large datasets, its simplicity can be advantageous when dealing with smaller lists. The algorithm’s straightforward implementation and intuitive approach make it a popular choice for beginners learning about sorting algorithms.
When it comes to efficiency, bubble sort may not be the optimal choice. However, it can still be a valuable tool for programmers and product managers who are looking for a simple and quick way to sort data sets. Understanding the fundamentals of bubble sort can help them make informed decisions and prioritize their work efficiently.
FAQ
What is bubble sort?
Bubble sort is a simple comparison-based sorting algorithm that arranges a string of numbers or elements in the correct order. It works by repeatedly comparing adjacent elements and swapping them if they are in the wrong order.
Is bubble sort efficient for sorting large datasets?
No, bubble sort is not efficient for sorting large datasets. Its time complexity is O(N^2), which means the time it takes to sort a list of size N is proportional to N squared.
Can bubble sort be used for sorting small datasets or nearly sorted datasets?
Yes, bubble sort can work well for small datasets or datasets that are already mostly sorted. Its simplicity makes it easy to understand and implement for such scenarios.
How can product managers use bubble sort?
Product managers can use bubble sort as a methodology for prioritizing work when faced with limited time and resources. It provides a simple and intuitive approach to ranking and organizing tasks.
What are some other sorting algorithms?
Some other popular sorting algorithms include selection sort, insertion sort, merge sort, quick sort, heap sort, and bucket sort. The choice of algorithm depends on the specific requirements of the sorting task.
How does bubble sort work?
Bubble sort works by repeatedly comparing adjacent elements in a list and swapping them if they are in the wrong order. This process continues until the list is sorted.
In which programming languages can bubble sort be implemented?
Bubble sort can be implemented in various programming languages such as C++, Java, Python, PHP, JavaScript, and C#. Each language may have its own syntax and specific implementation details, but the basic logic of the algorithm remains the same.
What is the time complexity of bubble sort?
The time complexity of bubble sort is O(N^2), where N represents the size of the list to be sorted.
What is the space complexity of bubble sort?
Bubble sort has a space complexity of O(1), meaning it does not require any additional memory beyond the input list itself. It performs in-place sorting.
What are the advantages of bubble sort?
Bubble sort is easy to understand and implement, making it a good choice for teaching sorting concepts. It is an in-place sorting algorithm and maintains the relative order of elements with the same key value.
What are the disadvantages of bubble sort?
Bubble sort is not efficient for large datasets due to its time complexity. It is a comparison-based sorting algorithm, which can limit its efficiency in certain scenarios.
What are the common applications of bubble sort?
Bubble sort is commonly used in computer graphics for polygon filling algorithms to detect and fix errors in nearly sorted arrays.
Why should I understand bubble sort?
Understanding bubble sort can help programmers and product managers make informed decisions about sorting tasks, prioritize work, and grasp fundamental sorting concepts.
One thought on “Understanding The Bubble Sort: Simple Sorting Explained”