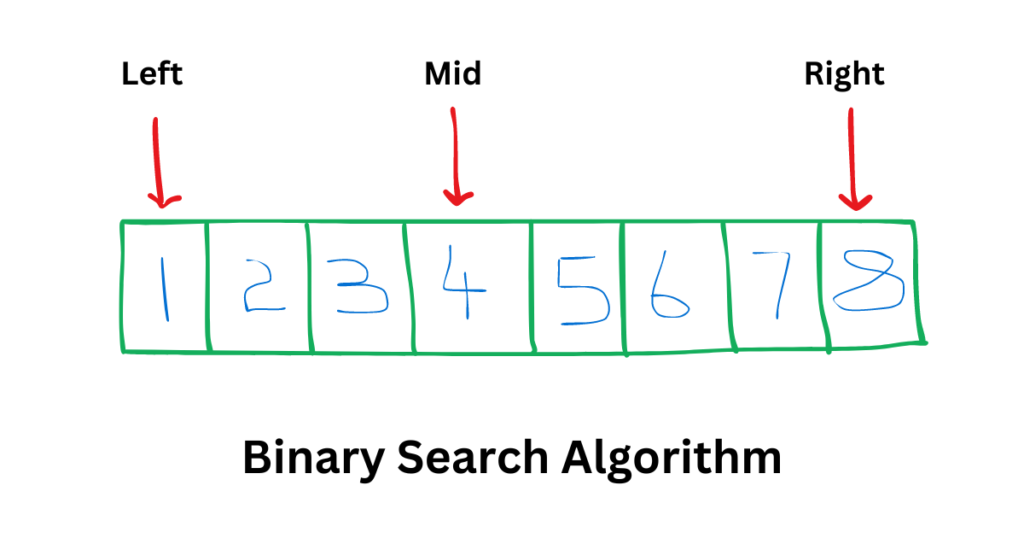
Table of Contents
Binary search is an efficient search algorithm used for finding elements in a sorted array. It follows a divide and conquer approach, making it a highly efficient and widely used search technique. By understanding its implementation and time complexity, we can optimize our search algorithms and improve overall efficiency.
When dealing with large datasets or when time is of the essence, binary search can be immensely helpful. It allows us to find the desired element in logarithmic time, ensuring a speedy and accurate search. This makes it significantly faster than linear search, especially in cases where the array is sorted.
Binary search works by repeatedly dividing the search space in half until the target element is found or the search space becomes empty. This process of dividing and conquering makes it efficient, as it eliminates half of the remaining elements with each iteration.
To better understand the time complexity of binary search, let’s consider some key points. In the best case scenario, the target element is found at the middle index, resulting in a time complexity of O(1), as it requires only one comparison. On the other hand, in the worst case scenario, where the target element is not present or located at the ends of the array, the time complexity is O(log N), where N is the size of the array.
Implementing binary search in various programming languages, such as Python, allows us to efficiently search for elements in sorted arrays. Understanding the time complexity and auxiliary space complexity of binary search is crucial for optimizing our search algorithms and improving overall efficiency in real-world applications.
How does binary search work?
Binary search is an algorithm used to efficiently search for elements in a sorted array. It works by dividing the search interval, which is the sorted array, into two halves. The algorithm starts by comparing the middle element of the array with the target element we are searching for.
If the middle element matches the target element, the search is complete, and the algorithm terminates. If the middle element is greater than the target element, the algorithm continues the search in the left half of the array. Conversely, if the middle element is smaller than the target element, the algorithm continues the search in the right half of the array.
This process of dividing the search interval in half and comparing the middle element with the target element continues recursively or iteratively until either the target element is found or the search interval becomes empty.
An important aspect of understanding how binary search works is through the use of a recurrence relation. The recurrence relation for binary search is represented as T(N) = T(N/2) + O(1), where N is the size of the array. This relation signifies that with each recursive or iterative step, the search interval is halved, resulting in a time complexity of O(log N), where N is the size of the array.
Time complexity of binary search
The time complexity of binary search is an important factor to consider when analyzing the efficiency of this search algorithm. It determines how the running time of binary search grows relative to the size of the array being searched.
In the best case scenario, when the target element is found at the middle index of the array, binary search has a time complexity of O(1). This means that it only requires one comparison to locate the desired element. This scenario represents the most efficient outcome for binary search, as the search terminates quickly.
On the other hand, in the worst case scenario, where the target element is not present in the array or is located at the ends of the array, the time complexity of binary search is O(log N), where N is the size of the array. This time complexity arises because, at each iteration of the binary search, the search space is divided in half, resulting in a halving of the remaining elements to be searched. This logarithmic growth in time complexity illustrates the efficiency of binary search in reducing the search space as the algorithm progresses.
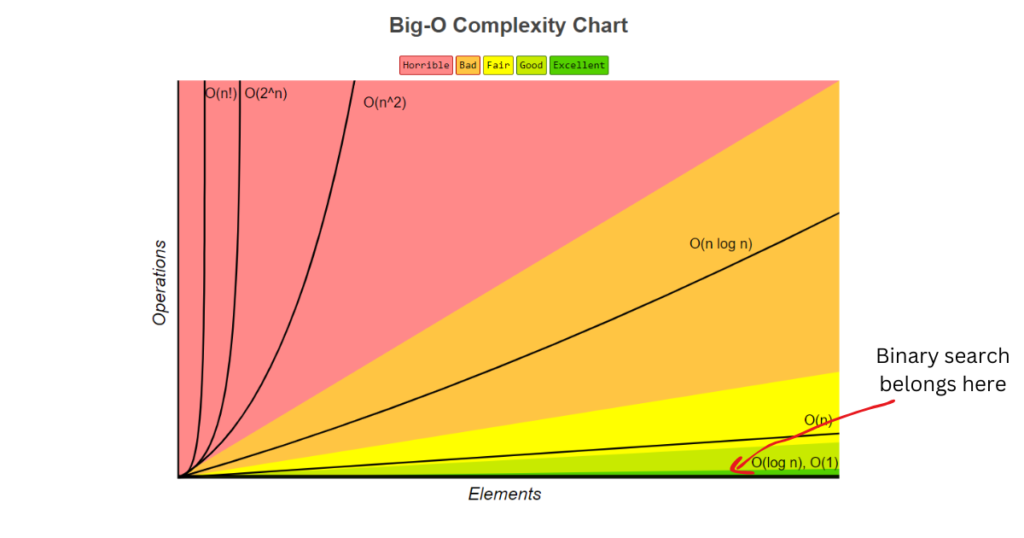
Image credits: Big O Cheat Sheet
On average, the time complexity of binary search is also O(log N). This average case time complexity takes into account all possible scenarios and provides a measure of the algorithm’s performance on average. The efficient divide and conquer approach of binary search ensures that the search space is significantly reduced with each iteration, leading to a logarithmic time complexity.
Understanding the time complexity of binary search is crucial for evaluating and optimizing search algorithms. By analyzing the best case, worst case, and average case scenarios, we can gain insights into the efficiency and performance of binary search in different situations.
Auxiliary space complexity of binary search
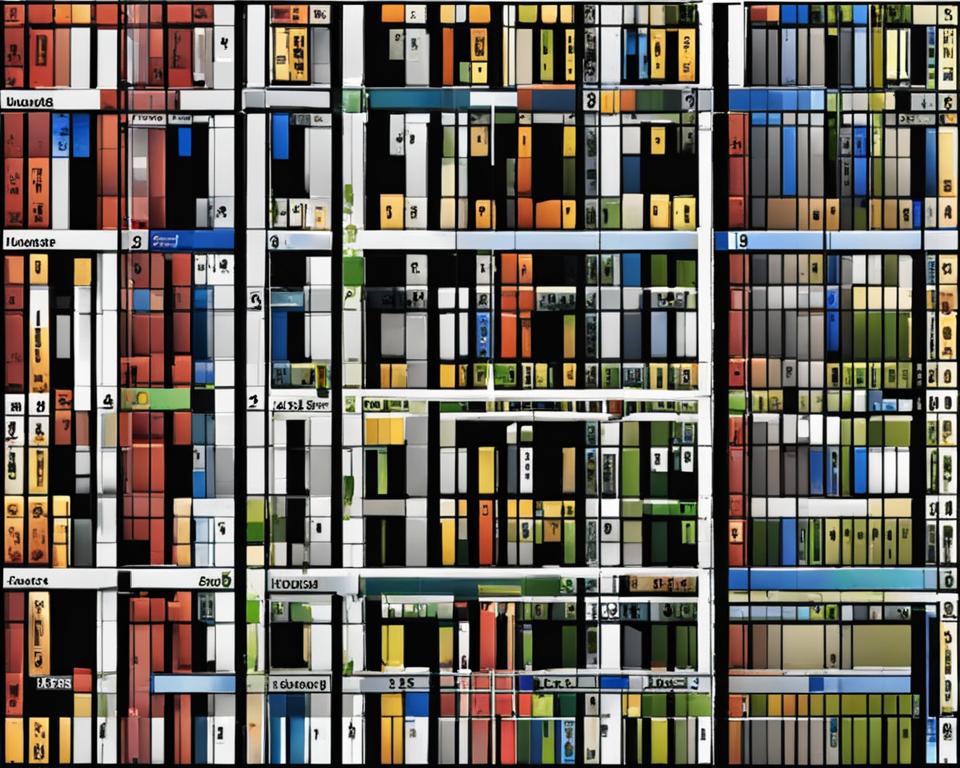
Binary search is a highly efficient algorithm that offers not only a fast time complexity but also an impressive auxiliary space complexity. When we talk about auxiliary space complexity, we are referring to the extra memory that an algorithm requires to perform its operations. In the case of binary search, it stands out for its minimal use of additional space.
Unlike other search algorithms that may require additional data structures or variables to execute their tasks, binary search operates with a constant amount of auxiliary space. This means that regardless of the size of the input array, binary search only needs a fixed amount of extra memory.
By utilizing a divide and conquer approach, binary search works by repeatedly halving the search space until it identifies the target element. In each iteration, it only requires a constant amount of additional space to store variables such as the middle index or the high and low indices. As a result, the auxiliary space complexity of binary search can be represented as O(1), indicating that it uses a constant amount of extra memory.
Visualization of Auxiliary Space Complexity
To better understand the auxiliary space complexity of binary search, let’s consider the following example:
In the visualization above, we can see how binary search efficiently utilizes its auxiliary space. As the search space is divided in half at each step, the additional memory required remains constant. This efficient use of resources contributes to the overall speed and performance of the binary search algorithm.
In summary, the auxiliary space complexity of binary search is O(1), indicating its ability to operate with a fixed amount of additional memory. This efficiency makes binary search an excellent choice for searching elements in a sorted array, especially when memory usage is a concern. By understanding and leveraging the auxiliary space complexity of binary search, developers can optimize their algorithms and improve overall efficiency.
How to implement binary search in Python
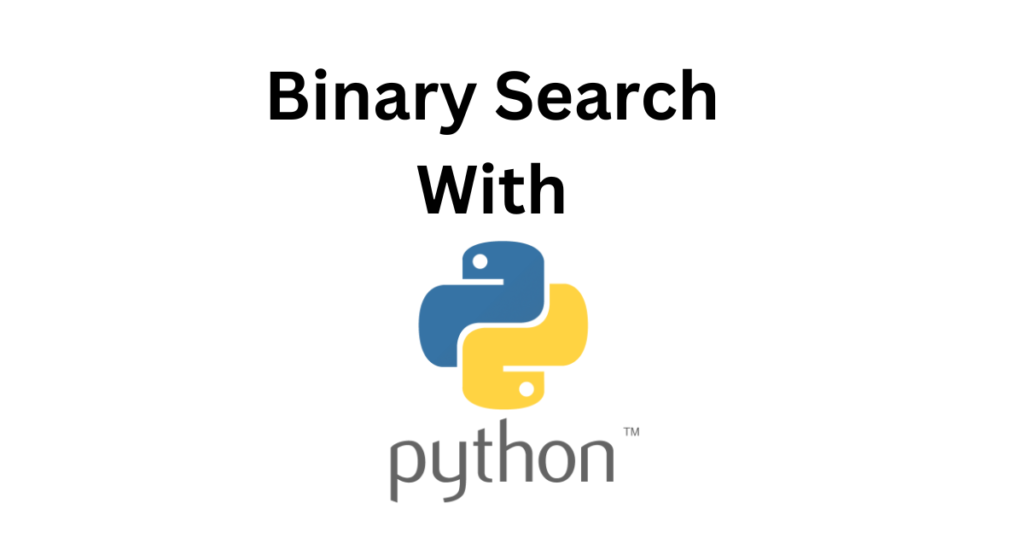
Binary search is a commonly used search algorithm that can be implemented in various programming languages, including Python.
Binary Search Function
To begin the Python implementation of binary search, we can define a function named binary_search. This function takes in an array and a target element.
The binary_search function compares the target element with the middle element of the current search space. If the target element is equal to the middle element, the function returns the index of the middle element, indicating a successful match. Otherwise, if the target element is less than the middle element, the function identifies the target is located below the middle element. Consequently, the function makes a boundary value of the right corner by assigning the right value to the middle value. On the other hand, if the target element is greater than the middle element, the function identifies the target is located above the middle element. Consequently, the function makes a boundary value of the left corner by assigning the left value to the middle value.
This results in a more efficient search, as the search space is divided in half at each step. Additionally, the use of low and high indices allows for better control over the search space boundaries.
Here is an example of the recursive binary search function in Python:
Time complexity of binary search in practice
In practice, binary search is widely utilized in numerous real-world applications that prioritize efficiency, especially when dealing with large datasets. The algorithm’s ability to efficiently search for records based on specific criteria makes it a popular choice in database systems.
One significant application of binary search is in algorithms for sorting and searching large collections of data. By employing the divide and conquer approach of binary search, these algorithms are able to achieve remarkable performance improvements compared to other search algorithms.
To better understand the impact of binary search in practice, consider the example of searching for a specific record in a database with millions of entries. By utilizing binary search, the algorithm can efficiently narrow down the search space with each iteration, significantly reducing the number of comparisons required. This leads to faster results, making binary search an optimal choice for optimizing search algorithms in scenarios involving large datasets.
By leveraging the efficiency and optimization capabilities of binary search, application developers and programmers can improve the overall performance and responsiveness of their systems, providing a seamless and efficient user experience. From sorting algorithms to searching for specific data points, binary search proves to be a valuable tool in various real-world scenarios.
Real-World Applications
Binary search finds its applications in a wide range of real-world scenarios, including:
- Database systems: Searching for records based on certain criteria.
- Sorting algorithms: Facilitating efficient sorting of large datasets.
- Online marketplaces: Searching for products based on specific attributes.
- Geolocation services: Finding points of interest within a specified range.
- Image recognition: Matching input images with a database of known images.
The real-world applications of binary search span across various industries, showcasing its versatility, efficiency, and ability to handle vast amounts of data. Whether it is to improve search performance, optimize algorithms, or enhance user experiences, binary search proves to be an invaluable tool in today’s data-driven world.
Find an element’s inserting position
By tweaking the binary search algorithm a little bit, we can come-up with an algorithm to find an element’s inserting position/index.
This algorithm is a two-in-one kind of solution. It not only searches the target index, but also it searches for the inserting index for a non-existing item in the input array.
Ex:- Provided input array is [3, 6, 8, 9] and number 7 is not available in the array. But the index number 7 should be added is 2 since number 6 is available in the array at index 1.
Conclusion
In summary, binary search is a highly efficient search algorithm that is particularly useful for sorted arrays. By dividing the search space in half at each step, binary search quickly narrows down the search range until the target element is found. This approach significantly reduces the time complexity of the search, making binary search much faster than linear search, especially for large arrays.
Implementing binary search in various programming languages, such as Python, allows for efficient searching in a wide range of applications. Whether it’s searching through a database system or sorting and searching large collections of data, the divide and conquer approach of binary search can greatly improve efficiency. By understanding the time complexity and auxiliary space complexity of binary search, developers can optimize search algorithms and enhance overall efficiency in their applications.
Overall, binary search is a powerful and versatile algorithm that offers significant advantages in terms of time complexity and efficiency. Its simplicity and effectiveness make it a key tool in the arsenal of every developer. By mastering the implementation and understanding the intricacies of binary search, developers can unlock its full potential and improve the performance of their search algorithms.
2 thoughts on “Implement binary search and calculating the time complexity”