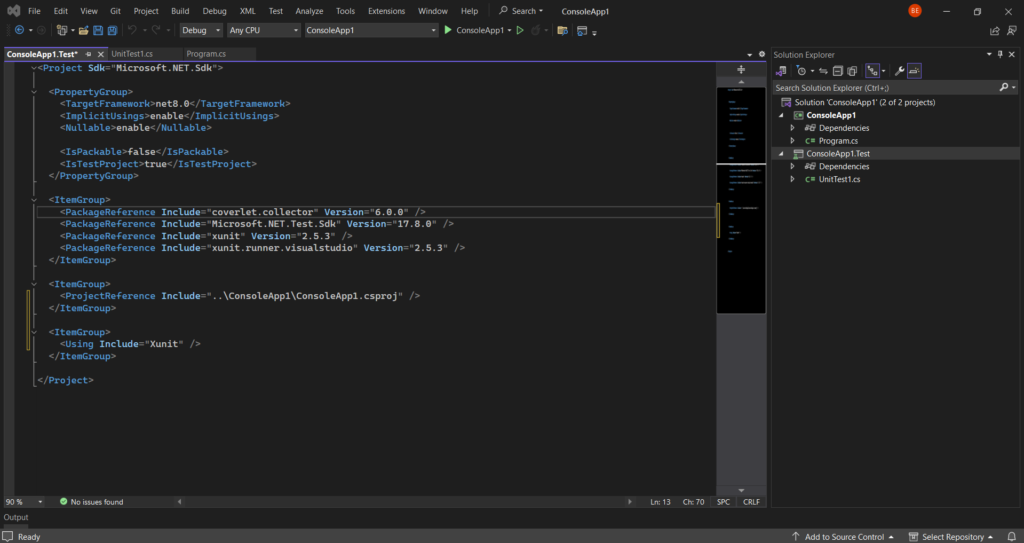
When we develop web or software applications as developers, writing unit tests is a crucial part of the process. This applies just as much to C#.NET application development as it does to any other programming language or framework.
Unit tests are small, self-contained code snippets that verify the correct behavior of individual units or components of an application. By testing these units in isolation, we can ensure that they function as intended and detect any potential bugs or issues early on in the development cycle.
In C#.NET application development, unit testing plays a vital role in maintaining code quality and preventing regressions. With proper unit tests in place, we can confidently make changes or updates to our codebase, knowing that we have a safety net of tests to catch any unexpected side effects.
This article will explore the importance of unit testing in C#.NET application development and provide best practices for implementing effective tests. We will also address common issues that developers may encounter and provide solutions to overcome them.
Table of Contents
Key Takeaways:
- Unit testing is crucial for ensuring the correct behavior of individual units or components in a C#.NET application.
- Proper unit tests help maintain code quality and prevent regressions.
- Following best practices, such as writing meaningful test names and testing for both positive and negative scenarios, enhances the effectiveness of unit testing.
- Common issues in C#.NET unit testing can be resolved by checking project references, choosing the correct test project template, and verifying the target framework versions.
- Regularly reviewing and updating tests is essential to reflect the current functionality of the codebase.
The naming convention for the unit tests project
[Project’s name planning to test].[UnitTests]
Let’s assume the project name is ConsoleApplication.
So the unit test project name should be ConsoleApplication.UnitTests.
Please note that all the following fixes and suggestions are based on the above naming convention.
C#.NET Unit Test Common issues and solutions
When setting up unit testing in a C# project with Xunit or Nunit, several common issues may arise. Here are some troubleshooting solutions:
- Test project not targeting the correct .NET version: Ensure that the test project’s target framework matches the main project’s .NET version. If the desired version is not available, try using a different test project template or update the test project’s target framework.
- Incorrect test framework references: Verify that the necessary NuGet packages for the chosen test framework (Xunit or Nunit) are installed in both the main project and the test project. Update the packages if necessary to ensure compatibility.
- Missing project references: Double-check that the main project is correctly referenced in the test project and that all required namespaces and classes are imported. If not, use the appropriate project reference command to add the necessary reference.
- Build configuration issues: Check that the build configuration for both the main project and the test project is properly set. Pay attention to multiple build configurations (e.g., Debug, Release) or platform targets (e.g., x86, x64) that may cause conflicts.
The most common issue, and fixes for it
The following error is a common one.
DynamicProxyGenAssembly2 System.ArgumentException : Can not create proxy for type because it is not accessible. Make it public, or internal and mark your assembly with [assembly: InternalsVisibleTo(“DynamicProxyGenAssembly2”)] attribute, because assembly is not strong-named. (Parameter ‘interfaceToProxy’)
The root cause for the above issue is in C#, classes are by default not ‘public’ to the outside the project’s assembly. They show as ‘internal’ classes. There are two main fixes for the above issue.
- Make all classes ‘public’ instead of ‘internal’.
- But the best practice is not making classes public. Below is the fix.
In the main project’s .csproj configurations should be updated with a new item group as below.
<ItemGroup>
<!-- Make assembly visible to test assembly -->
<AssemblyAttribute Include="System.Runtime.CompilerServices.InternalsVisibleTo">
<_Parameter1>$(AssemblyName).UnitTests</_Parameter1>
</AssemblyAttribute>
</ItemGroup>
And your unit test project’s .csproj configurations should be below after adding a project reference to the main project.
<ItemGroup>
<ProjectReference Include="..\[Main Project Path].csproj" />
</ItemGroup>
Testing best practices
When conducting unit testing in C# projects, it is crucial to follow these best practices to ensure efficient and effective testing:
- Write meaningful test names: Provide clear and descriptive names for your tests that accurately convey the scenario being tested and the expected behavior.
- Test all edge cases: To achieve comprehensive test coverage, make sure to cover a wide range of input combinations and edge cases, including both valid and invalid inputs.
- Use mocking and stubbing frameworks: When dealing with complex dependencies or external resources, leverage frameworks like Moq or NSubstitute to create mocks or stubs and facilitate isolated testing.
- Test for both positive and negative scenarios: Don’t just focus on testing the expected behavior; be sure to include tests for unexpected scenarios, error handling, and edge cases to validate the robustness of your code.
- Keep tests independent and isolated: Each test should not depend on the state or results of other tests. This isolation ensures that failures in one test do not impact the accuracy and reliability of other tests.
- Automate tests execution: Embrace continuous integration (CI) and continuous delivery (CD) practices to automatically run tests upon code changes and deployments, ensuring that tests are always up to date and executed consistently.
- Regularly review and update tests: As your codebase evolves, periodically review and update your tests to align with any changes in functionality, ensuring that your tests accurately reflect the current behavior of your code.
- Aim for high test coverage: Strive for a high percentage of test coverage to thoroughly validate critical code paths and maintain code quality. A comprehensive suite of tests helps identify potential bugs and reduces the risk of introducing regressions into the codebase.
By adhering to these best practices, you can enhance the reliability, maintainability, and overall quality of your C# unit testing efforts, leading to more robust and bug-free code.
Conclusion
In conclusion, troubleshooting issues related to unit testing in C# projects, particularly when using Xunit or Nunit, requires careful consideration of various factors such as project references, test project templates, and target framework versions. By following best practices in unit testing, we can ensure that our code is thoroughly tested, improving code quality and reducing the likelihood of bugs and errors.
Regularly reviewing and updating tests as the codebase evolves is crucial to maintain accurate and comprehensive test coverage. This practice ensures that our tests accurately reflect the current functionality of our code, keeping them relevant and effective.
With C# unit testing frameworks like Xunit and Nunit, developers have powerful tools at their disposal to simplify and streamline the testing process. By leveraging these frameworks and adhering to best practices, we can confidently troubleshoot and address any unit testing issues that arise, ultimately delivering high-quality, robust code.
One thought on “C#.NET project with Xunit/Nunit unit testing project referencing not working issue fix”